따로 오라클 디비가 없는 관계로 json-server를 통한 fake db를 구축할 예정이다
Json-Server 설치 및 사용법 (더보기↓)
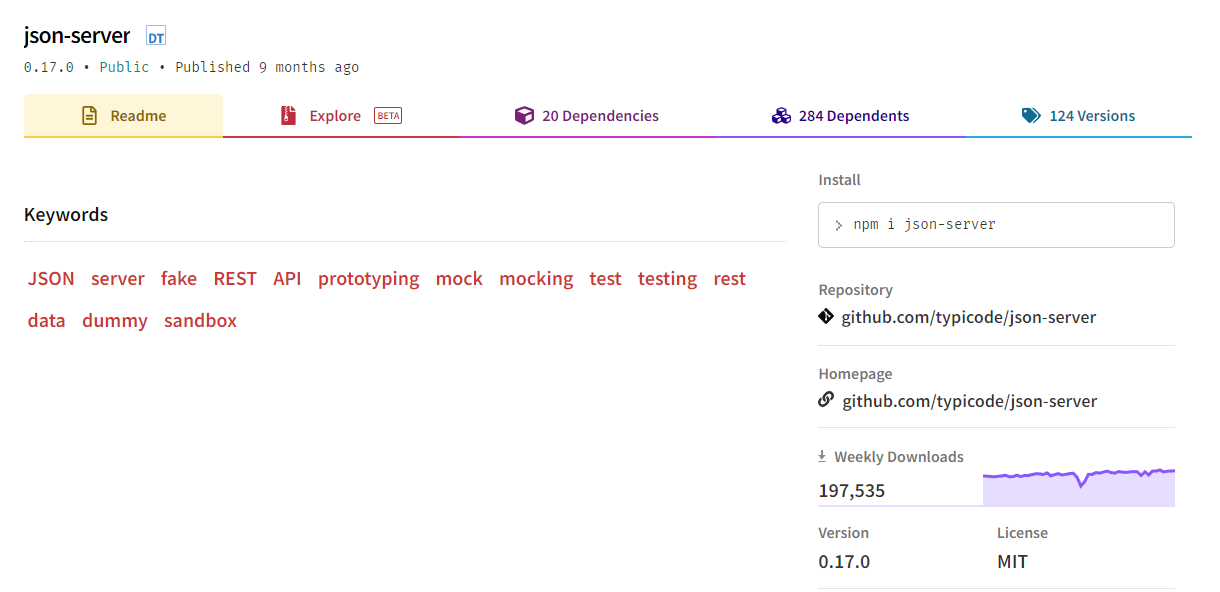
npm i json-server --save
설치가 다 되었다면 터미널에 실행 명령을 친다
json-server --watch db.json
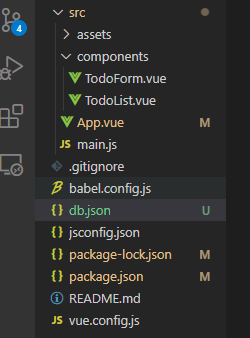

{
"posts": [
{ "id": 1, "title": "json-server", "author": "typicode" }
],
"comments": [
{ "id": 1, "body": "some comment", "postId": 1 }
],
"profile": { "name": "typicode" }
}
이렇게 적혀있다. 3000/post , 3000/comments ...
id, title,author 칼럼을 가진 posts라는 테이블명 이라고 생각하면 된다!
싹 지우고
{
"todolist": []
}
todolist 테이블 하나 넣어주고 ctrl + s (세이브) ^^~
동기 비동기를 사용하기 위해서 axios 모듈을 사용하기 때문에 axios를 import
npm install axios --save
import axios from "axios";
기존에 있던 todolist에 입력받은 todo값을 push하던 함수를 손본다.
var todoAddapp = (todo) => {
//db 저장
axios.post("http://localhost:3000/todolist", {
sbj: todo.sbj,
isdone: todo.isdone,
}).then(res=>{
todolist.value.push(res.data);
}).catch(err=>{
console.log(err);
});
};
axios.post("db주소",{
칼럼명1 : 내용(sbj =string)
칼럼명2 : 내용(isdone = boolean)
})
그뒤 response값을 console에 찍어보면 객체에 담긴 id,sbj,isdone 값(id는 json-server에서 자동으로 매겨줌)을 todolist에 push한다. 뒤 내용은 예외처리
이때 async&await을 사용하면 더 깔끔한 동기 처리가 가능하다
var todoAddapp = async (todo) => {
try {
//db 저장
let res = await axios.post("http://localhost:3000/todolist", {
sbj: todo.sbj,
isdone: todo.isdone,
});
todolist.value.push(res.data);
} catch (err) {
throw err;
}
};
async의 작동원리는 아직 학습이 미흡하여 제대로된 설명이 불가하지만, 하나의 공식이라 생각하기로 했다..
await axios값을 변수 (let res)로 받는다. 정도?
궁금하면 console.log(res), console.log(res.data); 를 찍어가며 res에 어떤 값이 들어오나 확인해보는것도 방법이다.
삭제, 조회 역시 동일하게 진행된다
App.vue 코드 전문 (더보기↓)
<template>
<div class="container">
<h1>TodoList</h1>
<input
class="form-control"
type="text"
v-model="search"
placeholder="검색"
/>
<hr />
<TodoForm @todoAdd="todoAddapp" />
<TodoList
:todolist="searchTodolist"
@toggle-todo="toggleTodo"
@delete-item="deleteitem"
/>
<div v-if="!todolist.length">
<b>오늘은 갓백수의 삶을 꿈꾸시나용..?</b>
</div>
</div>
</template>
<script setup>
import { ref, computed } from "vue";
import axios from "axios";
import TodoForm from "./components/TodoForm.vue";
import TodoList from "./components/TodoList.vue";
let todolist = ref([]);
let getTodolist = async () => {
try {
let res = await axios.get("http://localhost:3000/todolist");
todolist.value = res.data;
//console.log(res.data[0].sbj);
} catch (err) {
console.log(err);
}
};
getTodolist();
let search = ref("");
let searchTodolist = computed(() => {
if (search.value) {
return todolist.value.filter((tmp) => {
return tmp.sbj.includes(search.value);
});
} else {
return todolist.value;
}
});
var deleteitem = async (index) => {
let id = todolist.value[index].id;
try {
let res = await axios.delete("http://localhost:3000/todolist/" + id);
console.log(res);
// console.log(index)
} catch (err) {
throw err;
}
todolist.value.splice(index, 1);
};
//비동기로 만든다
var todoAddapp = async (todo) => {
try {
//db 저장
let res = await axios.post("http://localhost:3000/todolist", {
sbj: todo.sbj,
isdone: todo.isdone,
});
todolist.value.push(res.data);
} catch (err) {
throw err;
}
};
var toggleTodo = (index) => {
todolist.value[index].isdone = !todolist.value[index].isdone;
};
// let todoStyle = {
// textDecoration: "line-through",
// color: "gray",
// };
</script>
<style>
.todoStyle {
color: red;
text-decoration: line-through;
}
</style>
TodoForm.vue 코드전문 (더보기)
<template>
<form @submit.prevent="Submit" class="d-flex">
<div class="flex-grow-1">
<input
class="form-control"
type="text"
v-model="todo"
placeholder="할일"
/>
</div>
<div>
<button class="btn btn-primary">추가</button>
</div>
</form>
</template>
<script>
import { ref } from "vue";
export default {
setup(props, context) {
//props는 아직 잘 모르겠고 context가 부모로 보내는 부분
let todo = ref("");
var Submit = () => {
context.emit("todoAdd", {
id: Date.now(),
sbj: todo.value,
isdone: false,
}); //context.emit할때 보내려는 이름, {값들}
todo.value = "";
};
return {
todo, //여기있는 return은 app.vue와 마찬가지로 자식컴포넌트 내에서 사용되는 변수 및 함수명
Submit,
};
},
};
</script>
<style></style>
TodoList.vue 코드전문 (더보기)
<template>
<ul class="list-group" v-for="(i, index) in todolist" v-bind:key="i.id">
<li class="list-group-item d-flex align-items-center mt-2">
<div class="form-check flex-grow-1">
<input
class="CheckInput"
:value="todolist.isdone"
@change="toggleTodo(index)"
type="checkbox"
/>
<label class="checkLabel" :class="{ todoStyle: i.isdone }"
>{{ i.sbj }}
</label>
</div>
<div>
<button class="btn btn-danger btn-sm" @click="deleteitem(index)">
삭제
</button>
</div>
</li>
</ul>
</template>
<script setup>
// import { defineProps, defineEmits } from "vue";
const props = defineProps({
todolist: {
type: Array,
required: true,
},
});
const emit = defineEmits(["toggle-todo", "delete-item"]);
const toggleTodo = (index) => {
emit("toggle-todo", index);
};
const deleteitem = (index) => {
emit("delete-item", index);
};
//props:['todolist']//받아오는 이름 + 위에서 사용하는 이름 props는 return역할을 함께함
</script>
<style></style>
'FrontEnd > Vue3' 카테고리의 다른 글
Vue3 Timeout() [JavaScript] (0) | 2022.07.05 |
---|---|
Vue3 To do list 만들기 (10) Pagination , WatchEffect (0) | 2022.06.28 |
Vue3 To do list 만들기 (8) 검색기능 추가하기 (0) | 2022.06.24 |
Vue3 To do list 만들기 (7) props 주의사항 (0) | 2022.06.24 |
Vue3 To do list 만들기 (6) props 부모->자식 데이터 전송 (0) | 2022.06.23 |